Dynamically creation of web controls and binding Validations Using ASP.NET
Dynamically creation of web controls and binding Validations Using ASP.NET
Source Code : creatingdynamiccontrols2.zip
Introduction:-
As we know we can bind the controls to our page (aspx) in 2 ways
1. Statically :- Binding the controls while Designing
2. Dynamically :- Binding the controls at runtime
Everyone know how to bind statically but there may be some cases where we need to bind the control dynamically .One of those cases is when our controls are keep on changing like I have a registration site in which the control type may keep on changing then I may need to go for binding the controls dynamically .
Approach:
For binding the controls I need the data what are the controls I want to bind and what is the id to be given and what are the properties to be provided etc.,
This can be provided by 2 ways
1. Using database (This is my approach)
2. Using Xml file
I am creating one example (registration Page) where I am getting the data from the database as a table and based on this table I will be binding my controls to the page as we can see below
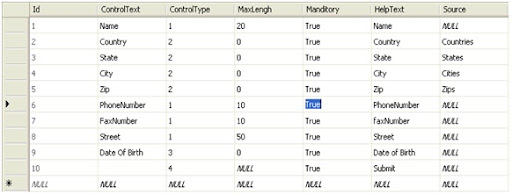
My Controls Data from database
ControlText : - Text to be Displayed
ControlType :-
1 – TextBox Control
2 – DropDown Control
3 – DateTime Control
4 – Buttom Control
MaxLength:- Defining the maximum length for textbox
Manditory :- Based On this we will be binding the required field validation
HelpText :- This is used to bind as ToolTip for the control
Source :- Use for binding data source for dropdown controls
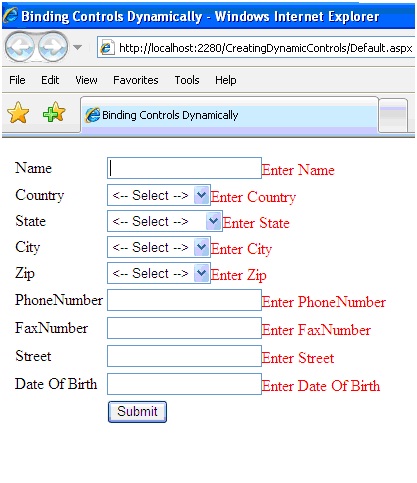
Page in which all the controls are generated dynamically
Implementation:
As we know all the server control are under System.Web.UI.WebControls namespace
I will be getting the tabel into a dataset for suppose the name is controlsDataSet
///
/// Method usd to bind the controls
///
///
private void BuldControls(DataSet controlsDataSet)
{
Table table = new Table();
table.Style.Add("align", "center");
// Looping the dataset and get the values
foreach (DataRow datarow in controlsDataSet.Tables[0].Rows)
{
int controlType =
Convert.ToInt32(datarow["ControlType"].ToString());
string controlText = datarow ["ControlText"].ToString();
TableRow newRow = new TableRow();
TableCell textCell = new TableCell();
// Binding the text
textCell.Text = controlText;
newRow.Cells.Add(textCell);
TableCell valueCell = new TableCell();
Control[] cntrlCollection=new Control[4];
// Checking the control Type
switch(controlType)
{
// If TextBox
case 1:
cntrlCollection = this.BindTextBox(control);
break;
// If DropDown
case 2:
cntrlCollection = this.BindDropDown(control);
break;
// If DateTime
case 3:
cntrlCollection = this.BindDateTime(control);
break;
// If Button
case 4:
cntrlCollection = this.BindButton(control);
break;
}
// Adding th controls to the table cell
foreach (Control cntrl in cntrlCollection)
{
if (cntrl != null)
{
valueCell.Controls.Add(cntrl);
}
}
newRow.Cells.Add(valueCell);
table.Rows.Add(newRow);
}
// Adding the table to the main panel
this.pnlControls.Controls.Add(table);
}
Creating controls dynamically is not a big matter but we have bind in such a way that when we are retriving it should not be a problem so we need to give the ids of the controls as below
///
/// Method to bind the DropDown
///
///
///
private Control[] BindTextBox(DataRow datarow)
{
// Retriving the values from the datarow
int controlId = Convert.ToInt32(datarow["Id"].ToString());
int length = Convert.ToInt32(datarow["MaxLengh"].ToString());
bool required =
Convert.ToBoolean(datarow["Manditory"].ToString());
string helpText = datarow["HelpText"].ToString();
string controlText = datarow["ControlText"].ToString();
// Creating the controls array
Control[] controls = new Control[4];
// creating the textbox
TextBox textbox = new TextBox();
textbox.ID = string.Concat(“txt”, controlId);
textbox.ToolTip = helpText;
// Checking the length
if (length != 0)
{
textbox.MaxLength = length;
}
// Adding the textbox to the control array
controls[0] = textbox;
// Checking id manditory
if (required)
{
controls[1] = this.BindRequiredvalidator(controlId,
textbox.ID, controlText);
}
return controls;
}
Suppose I am bind for the name column the textbox id becomes like this
“txt_1” and while saving also we can save accordingly.
So this is the way how to bind the controls and now coming to validation controls (I and using the controls provided my the VS).I am showing you a example where I am bind a validation control to textbox as shown below
private RequiredFieldValidator BindRequiredvalidator(int Id, string
controlId, string controlText)
{
RequiredFieldValidator requiredFieldValidator = new
RequiredFieldValidator();
requiredFieldValidator.ID = string.Concat(“rfv”, Id);
requiredFieldValidator.ControlToValidate = controlId;
requiredFieldValidator.SetFocusOnError = true;
requiredFieldValidator.ErrorMessage = string.Concat(“Enter “,
controlText);
return requiredFieldValidator;
}
For this method I will be passing the Id , ControlId (Like textbox control id) which need to be validated ,controlText used for error messaeges
And after doing all these we can see the paeg with validation controls like this
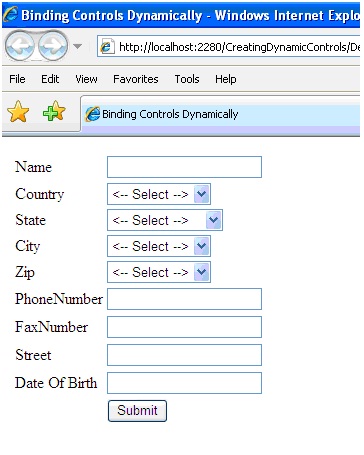
With Validation controls
Advantages over binding controls statically:
1. Suppose we need to add 10 controls and we are using those statically but now I need to change my control type like a textbox to be changed to dropdown then I need to change all my code once again but this easier while binding controls dynamically.
2. Can bind any validation to any control dynamically.
Source Code : creatingdynamiccontrols2.zip
Introduction:-
As we know we can bind the controls to our page (aspx) in 2 ways
1. Statically :- Binding the controls while Designing
2. Dynamically :- Binding the controls at runtime
Everyone know how to bind statically but there may be some cases where we need to bind the control dynamically .One of those cases is when our controls are keep on changing like I have a registration site in which the control type may keep on changing then I may need to go for binding the controls dynamically .
Approach:
For binding the controls I need the data what are the controls I want to bind and what is the id to be given and what are the properties to be provided etc.,
This can be provided by 2 ways
1. Using database (This is my approach)
2. Using Xml file
I am creating one example (registration Page) where I am getting the data from the database as a table and based on this table I will be binding my controls to the page as we can see below
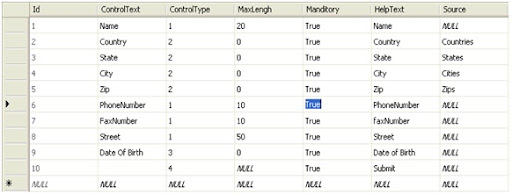
My Controls Data from database
ControlText : - Text to be Displayed
ControlType :-
1 – TextBox Control
2 – DropDown Control
3 – DateTime Control
4 – Buttom Control
MaxLength:- Defining the maximum length for textbox
Manditory :- Based On this we will be binding the required field validation
HelpText :- This is used to bind as ToolTip for the control
Source :- Use for binding data source for dropdown controls
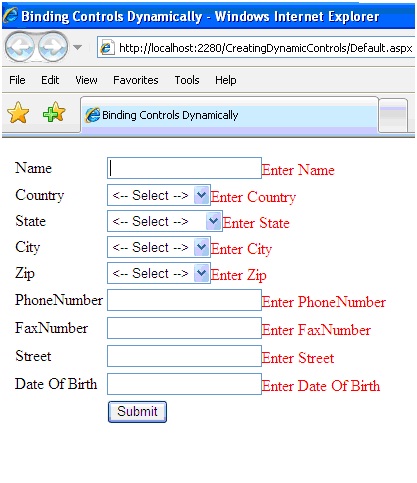
Page in which all the controls are generated dynamically
Implementation:
As we know all the server control are under System.Web.UI.WebControls namespace
I will be getting the tabel into a dataset for suppose the name is controlsDataSet
///
/// Method usd to bind the controls
///
///
private void BuldControls(DataSet controlsDataSet)
{
Table table = new Table();
table.Style.Add("align", "center");
// Looping the dataset and get the values
foreach (DataRow datarow in controlsDataSet.Tables[0].Rows)
{
int controlType =
Convert.ToInt32(datarow["ControlType"].ToString());
string controlText = datarow ["ControlText"].ToString();
TableRow newRow = new TableRow();
TableCell textCell = new TableCell();
// Binding the text
textCell.Text = controlText;
newRow.Cells.Add(textCell);
TableCell valueCell = new TableCell();
Control[] cntrlCollection=new Control[4];
// Checking the control Type
switch(controlType)
{
// If TextBox
case 1:
cntrlCollection = this.BindTextBox(control);
break;
// If DropDown
case 2:
cntrlCollection = this.BindDropDown(control);
break;
// If DateTime
case 3:
cntrlCollection = this.BindDateTime(control);
break;
// If Button
case 4:
cntrlCollection = this.BindButton(control);
break;
}
// Adding th controls to the table cell
foreach (Control cntrl in cntrlCollection)
{
if (cntrl != null)
{
valueCell.Controls.Add(cntrl);
}
}
newRow.Cells.Add(valueCell);
table.Rows.Add(newRow);
}
// Adding the table to the main panel
this.pnlControls.Controls.Add(table);
}
Creating controls dynamically is not a big matter but we have bind in such a way that when we are retriving it should not be a problem so we need to give the ids of the controls as below
///
/// Method to bind the DropDown
///
///
///
private Control[] BindTextBox(DataRow datarow)
{
// Retriving the values from the datarow
int controlId = Convert.ToInt32(datarow["Id"].ToString());
int length = Convert.ToInt32(datarow["MaxLengh"].ToString());
bool required =
Convert.ToBoolean(datarow["Manditory"].ToString());
string helpText = datarow["HelpText"].ToString();
string controlText = datarow["ControlText"].ToString();
// Creating the controls array
Control[] controls = new Control[4];
// creating the textbox
TextBox textbox = new TextBox();
textbox.ID = string.Concat(“txt”, controlId);
textbox.ToolTip = helpText;
// Checking the length
if (length != 0)
{
textbox.MaxLength = length;
}
// Adding the textbox to the control array
controls[0] = textbox;
// Checking id manditory
if (required)
{
controls[1] = this.BindRequiredvalidator(controlId,
textbox.ID, controlText);
}
return controls;
}
Suppose I am bind for the name column the textbox id becomes like this
“txt_1” and while saving also we can save accordingly.
So this is the way how to bind the controls and now coming to validation controls (I and using the controls provided my the VS).I am showing you a example where I am bind a validation control to textbox as shown below
private RequiredFieldValidator BindRequiredvalidator(int Id, string
controlId, string controlText)
{
RequiredFieldValidator requiredFieldValidator = new
RequiredFieldValidator();
requiredFieldValidator.ID = string.Concat(“rfv”, Id);
requiredFieldValidator.ControlToValidate = controlId;
requiredFieldValidator.SetFocusOnError = true;
requiredFieldValidator.ErrorMessage = string.Concat(“Enter “,
controlText);
return requiredFieldValidator;
}
For this method I will be passing the Id , ControlId (Like textbox control id) which need to be validated ,controlText used for error messaeges
And after doing all these we can see the paeg with validation controls like this
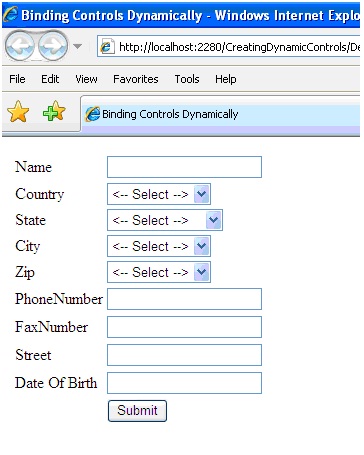
With Validation controls
Advantages over binding controls statically:
1. Suppose we need to add 10 controls and we are using those statically but now I need to change my control type like a textbox to be changed to dropdown then I need to change all my code once again but this easier while binding controls dynamically.
2. Can bind any validation to any control dynamically.
Comments
Post a Comment